WPF stands for Windows Presentation Framework and we are going to see how quick it is to utilize Esri’s Mobile 10 SDK’s new ability to work in WPF. In this blog I am not going to go into depth about WPF except to say it is Microsoft’s (MS) equivalent to Adobe AIR. Say goodbye to WinForms and welcome to a whole range of slick animations, affects and all that other jazzy stuff you now expect from any Silverlight or Flex web applications. But with this blog we are just going to focus on the nuts and bolts of quickly standing up a mobile application designed for a tablet that runs a Windows operating system. Plus I will point out some gotchas I stumbled across along the way.
Prerequisites:
- MS Visual Studio 2008 sp1 or higher (I personally prefer 2010- more built in features I make use of)
- Esri Mobile 10 SDK (make sure and run the uninstaller application first to identify any conflicts with prior installed versions of ArcGIS products)
- ArcGIS 10 (optional- but need if you want to use geoprocessing tools to create mobile caches)
- Created mobile caches
- Running mobile map service (unless you want to skip the sync part)
Map Tutorial Application
Once the prerequisites are installed, open Visual Studio and create a new project. In the new project dialog, select the WPF Windows Application template. For this tutorial, I chose to go down the path of C# (sorry you VB.NET programmers- but just Google a translator. None of the code snippets will be too difficult to translate). Name your new project WpfMobileTutorial (or whatever you want) and click OK. When your new project solution opens you will see two files- App.xaml and MainWindow.xaml. Expanding them and you see their corresponding cs files. For this tutorial we will ignore the App.xaml file. The only thing I will point out is in App.xaml you can set what xaml file in your project is the start up window- StartupUri=”MainWindow.xaml”
Now let’s pull in our Mobile SDK. Right click References folder and select to Add References. Add ESRI.ArcGIS.Mobile. If you had used Mobile 9.3.1 SDK or earlier you are probably used to going to the Toolbox window and seeing your Esri controls like map. There is a bug in the current release of this SDK and you will not see them in Toolbox but don’t despair- there are there. We just need to add them the old fashion way before we had fancy dandy IDEs that did all that work for us. First add the reference to the SDK to the Window header tag like so:
<Window x:Class="WpfMobileTuturial.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml" xmlns:esrimap="clr-namespace:ESRI.ArcGIS.Mobile.WPF;assembly=ESRI.ArcGIS.Mobile"
Title="MainWindow" Height="450" Width="650">
Add the following line inside your Grid container.
<Grid>
<esrimap:Map x:Name="map1" />
</Grid>
You could compile and launch but nothing fun will happen- we need to add the cache to the map. Now, I have done Flex development and I love the config files they use so things can be changed without recompiling. So, I mimic that by adding an xml file to my project that I call Settings.xml. You can setup how you want but this is the format I typically use to store my cache information:
<?xml version="1.0" encoding="utf-8" ?>
<configuration>
<cacheLocations>
<operationalCacheLocation>C:\Caches\OperationalCache\MobileCache</operationalCacheLocation>
<baseMapCacheLocation>C:\Caches\BaseMapCache\Layers</baseMapCacheLocation>
</cacheLocations>
<mobileServiceInfo>
<mobileServiceURL>http://serverTutorial/ArcGIS/services/Panhandle/tutorial/MapServer/MobileServer</mobileServiceURL>
</mobileServiceInfo>
</configuration>
For now let’s focus on what is between the cacheLocations tags. Most mobile applications will have separate caches. One for the stuff they plan to edit and sync- operationCacheLocation tag. And the base stuff used for reference and not typically synced- baseMapCacheLocation tag. OK- back in MainWindow.xaml add the following event to the Window header tag Loaded=”Window_Loaded”. Switch over to MainWindow.xaml.cs and add the following code:
MobileCache mobileCache;
private void Window_Loaded(object sender, RoutedEventArgs e)
{
string xmlPath = getAppPath() + "Settings.xml";
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(xmlPath);
XmlNodeList elemList;
}
public static bool IsDesignMode()
{
return (System.Diagnostics.Debugger.IsAttached);
}
public static string getAppPath()//make sure return with trailing \
{
bool check = IsDesignMode();
if (check)
{
return @"C:\Projects\WpfMobileTuturial\WpfMobileTuturial\";
}
else
{
return System.IO.Path.GetDirectoryName(System.Reflection.Assembly.GetExecutingAssembly().Location) + @"\";
}
}
private MobileCache getMobileCache(String cachePath)
{
MobileCache m_mobileCache = new MobileCache();
m_mobileCache.StoragePath = cachePath;
m_mobileCache.Open();
return m_mobileCache;
}
Let’s go over what is going on here. First, if you had done mobile development prior to 10 MobileServices was replaced with MobileCache. But be warned- it is not an exact switch out between them. So, I would try and forgot how MobileServices worked in 9.3.1 and treat MobileCache as something new you are learning. The helper methods getAppPath() and IsDesignMode() just do some of the leg work of finding where Settings.xml is- if debugging I did go ahead and hardcode the path but if it is installed I just pull out it of Assembly. I will discuss getMobilCache later. Let’s now add the code to our Window_Loaded method for adding the caches after the line XmlNodeList elemList;
elemList = xmlDoc.GetElementsByTagName("baseMapCacheLocation");
string streetCache = elemList[0].InnerXml;
if (streetCache != null && streetCache.Length > 0)
{
//rasterdata- local Tile Map Cache
TileCacheMapLayer mpLayer = new TileCacheMapLayer(streetCache);
mpLayer.Name = "Base Tile Cache";
mpLayer.Open();
map1.MapLayers.Add(mpLayer);
}
elemList = xmlDoc.GetElementsByTagName("operationalCacheLocation");
string localCache = elemList[0].InnerXml;
if (localCache != null && localCache.Length > 0)
{
mobileCache = getMobileCache(localCache);
//update the cache
elemList = xmlDoc.GetElementsByTagName("mobileServiceURL");
string mapurl = elemList[0].InnerXml;
int index = 0;
//map1.MapLayers.AddRange(mobileCache);
//this method AddRange doesn't always behave as expected so here is my work around- make a renderable mobilcachemaplayer for each layer
//and then add to WPF map
ReadOnlyLayerCollection clc = mobileCache.Layers;
int iCnt = mobileCache.Layers.Count;
for (int i = 0; i < iCnt; i++)
{
Layer layer = mobileCache.Layers[i];
MobileCacheMapLayer mobileCacheMapLayer = new MobileCacheMapLayer((MobileCacheLayer)layer);
map1.MapLayers.Insert(index, mobileCacheMapLayer);
index++;
}
}
So, all this code snippet does is first get the value from our tag baseMapCacheLocation and uses one of the new features of 10;using Tile Caches created from a Tile Map Service. You just need to copy _alllayers folder, conf.cdi and conf.xml locally and just have your Settings.xml store where they are saved locally.
The next part adds the operational layers to the MobileCache. And now you see us utilize the getMobileCache helper method. Just pass in the path location grabbed out of the Settings.xml and all this method does is initialize the mobile cache. Next we add these layers to the map. Here you will notice some comments in the code. I have had very inconsistent luck with using the map’s method AddRange. To be safe I always do the long way of casting the layers into MobileCacheMapLayer and add that to the map. Feel free to do whichever.
At this point you can compile and run and see a new window open showing your cache data..

Cool. Now let’s add some navigation. In MainWindow, drag three button controls onto your window and position where you would like them. Here you could get real fancy and add in some wicked style/animation affects. What I will do is just change text to Pan, Zoom In, and Zoom Out respectfully. I know, lame, but you can stop here and play with styling and if you have Expression Blend it is even easier. Here is a cool one to try- http://www.codeproject.com/KB/WPF/glassbuttons.aspx.
But back to the boring stuff let’s add the tiny bit of code snippets for adding in basic navigation:
private void btnPan_Click(object sender, RoutedEventArgs e)
{
map1.CurrentNavigationMode = ESRI.ArcGIS.Mobile.WPF.NavigationMode.Pan;
}
private void btnZoomIn_Click(object sender, RoutedEventArgs e)
{
map1.CurrentNavigationMode = ESRI.ArcGIS.Mobile.WPF.NavigationMode.ZoomIn;
}
private void btnZoomOut_Click(object sender, RoutedEventArgs e)
{
map1.CurrentNavigationMode = ESRI.ArcGIS.Mobile.WPF.NavigationMode.ZoomOut;
}
And hooking our WPF button controls to our methods add Click events to each of your buttons and point to corresponding method (e.g. Click=”btnPan_Click”). Now compile and run and you can navigate around your map by panning and zooming in and out.

Since this is a mobile application the last thing I will cover here is synchronization- especially since the process changed in 10. Let’s add a fourth button to our application. Again, I am not going to utilize any wicked cool affects and just change the text to ‘Sync’. And put the following method in MainWindow.xaml.cs:
private void btnSync_Click(object sender, RoutedEventArgs e)
{
try
{
string xmlPath = getAppPath() + "Settings.xml";
XmlDocument xmlDoc = new XmlDocument();
xmlDoc.Load(xmlPath);
XmlNodeList elemList;
elemList = xmlDoc.GetElementsByTagName("mobileServiceURL");
string url = elemList[0].InnerXml;
MobileServiceConnection mobileServiceConnection1 = new MobileServiceConnection();
//link the mobile classes to put the data into the display
mobileServiceConnection1.Url = url;
ReadOnlyLayerCollection clc = mobileCache.Layers;
int iCnt = mobileCache.Layers.Count;
for (int i = 0; i < iCnt; i++)
{
//synch just be feature test
FeatureLayer featureLayer = mobileCache.Layers[i] as FeatureLayer;
FeatureLayerSyncAgent featLayerSync = new FeatureLayerSyncAgent(featureLayer, mobileServiceConnection1);
FeatureLayerSyncResults featLayerResults = new FeatureLayerSyncResults();
featLayerResults = featLayerSync.Synchronize() as FeatureLayerSyncResults;
IDictionary<int, string> synErrors = featLayerResults.UploadedFeaturesErrors;
if (synErrors.Count > 0)
{
foreach (KeyValuePair<int, string> kvp in synErrors)
{
int v1 = kvp.Key;
string v2 = kvp.Value;
System.Windows.MessageBox.Show("Error uploading " + featureLayer.Name + ". Contact administrator. " + "Key: " + v1.ToString() + " Value: " + v2);
}
}
if (featLayerResults.Exception != null)
{
Exception e = featLayerResults.Exception;
if (e.Message != null && e.Message.Length > 0)
{
System.Windows.MessageBox.Show("Error uploading " + featureLayer.Name + ". Contact administrator. " + "Message: " + e.Message);
}
}
}
return;
}
catch (Exception e)
{
System.Windows.MessageBox.Show(e.Message, "Error in OpenCacheConnection", System.Windows.MessageBoxButton.OK, System.Windows.MessageBoxImage.Error);
return;
}
Looking at the code you now see the mobileServiceURL tag come into play. The URL to the mobile map service I also like to put in the Settings.xml. This, makes moving things to another environment much easier. Once we pull out the URL we assign to MobileServiceConnection. Now there was something I noticed about the sync process that annoyed me- it will run and not return any errors automatically. You actually have to dive into either FeatureLayerSyncResults or MobileCacheResults. In the above code snippet I actually show how to loop through cache and sync each layer. Then pull out if there were any errors in UploadedFeaturesErrors or in Exception. Makes debugging sync issues so much easier. So, go ahead and compile again and if you have your mobile service set up go ahead and sync. If there are any errors you will get an alert stating the problem.
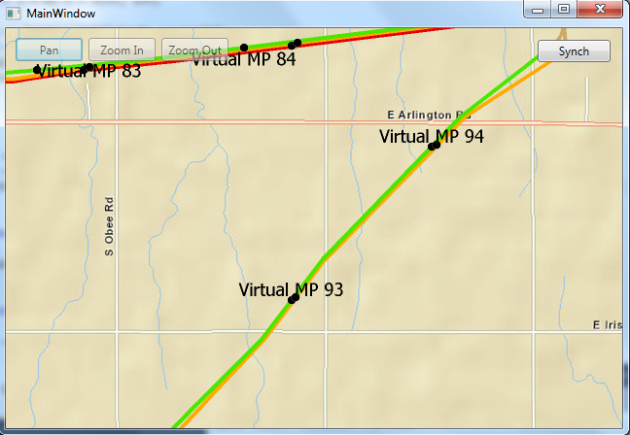
So, that ends the tutorial for getting up and running with Mobile 10 and WPF. I will be following this with a blog extending this tutorial to start incorporating aspects of the design framework MVVM and utilizing WPF binding capabilities. Also, if you would like to see how cool WPF can look check out our project showcase (http://showcase.gisinc.com/) and look for the Panhandle Energy Pilot Patrol Application or City of Appleton Sign Retro-reflectivity Solution projects.

No comments:
Post a Comment